Create menus for admin plugin
Updated at 1708699684000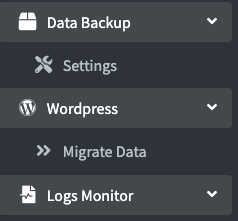
Creating Menus
Suppose we have a plugin called book-store
, and we want to have some menus:
- List of books.
- List of authors.
We can open the file book-store-admin-plugin/menus.properties
and add the following content:
extensions.book_store=/book-store extensions.book_store.books=/book-store/books extensions.book_store.authors=/book-store/authors
Where:
extensions
: Is the menu display area name.book_store
: Is the name of the parent menu, usually takes the form of the plugin's underscored name./book-store
,/book-store/books
,/book-store/author
: Are the exact URIs of the pages displayed when users click on the menu.
After export
, build
, and rerun BookStoreAdminPluginStartupTest
, you will see the menus displayed at the bottom of the sidebar as follows:
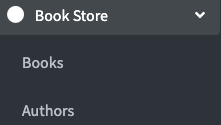
To better identify the menus, we can add icons to them by searching for free font icons from Font Awesome version 5 or Ionicons v2.
Suppose we find some favorite icons and update the contents of the menus.properties
file as follows:
extensions.book_store=/book-store; fas fa-book extensions.book_store.books=/book-store/books; fas fa-list extensions.book_store.authors=/book-store/authors; fas fa-users
After export
, build
, and rerun BookStoreAdminPluginStartupTest
, you will see the menus displayed at the bottom of the sidebar as follows:
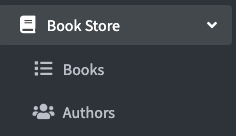
It looks much better, doesn't it?
Menu Display Areas
- top: Located at the top just below the media menu.
- components: Located behind the top menus, currently containing menus such as
Plugins
,Themes
,Socket App
. This is the area specialized in managing menus for administering components in EzyPlatform. - administrator: Located behind the components menus, currently containing menus such as
Admins
,Users
,Settings
. This is the area specialized in managing special administration menus such as users, administrators, or overall settings. - extensions: Located behind the administrator menus and before the
Logout
menu. This area is for plugins that are less frequently used by users.
For areas 1, 2, and 3, they are usually for plugins created by Young Monkeys or plugins commonly used by users. When you use these 3 areas, we will review very carefully to ensure you do not misuse the display position to provide the best experience for users.
The Book store is an important plugin for users to manage book-selling websites, so we will put it at the top by changing the extension
to top as follows:
top.book_store=/book-store; fas fa-book top.book_store.books=/book-store/books; fas fa-list top.book_store.authors=/book-store/authors; fas fa-users
After export
, build
, and rerun BookStoreAdminPluginStartupTest
, you will see the menus of book-store
displayed at the top of the sidebar.
Menu Items
EzyPlatform currently only provides single-level menus, as you can see after the plugin name Book Store
there is only one level to display the menus. Suppose you need second-level menus such as:
- Add a book.
- Book details.
- Update a book.
You can update the menus.properties
file as follows:
top.book_store=/book-store; fas fa-book top.book_store.books=/book-store/books; fas fa-list top.book_store.books_add=~/book-store/books/add top.book_store.books_details=~/book-store/books/{id} top.book_store.books_edit=~/book-store/books/{id}/edit top.book_store.authors=/book-store/authors; fas fa-users
You can see that the menu items will have a ~
before the URI and do not need to have an icon.
After export
, build
, and rerun BookStoreAdminPluginStartupTest
, you will see that the menus of the book-store
have not changed because the menu items will not be displayed on the interface.
Multilanguage
By default, when displaying menu names, they will be converted to capitalized and spaced, for example, books_add
will be displayed as Books Add
, which doesn't look very natural. You can change them by adding to language files, for example:
book-store-admin-plugin/src/main/resources/messages/messages.properties
books_add=Add Book books_details=Book Details books_edit=Edit Book
book-store-admin-plugin/src/main/resources/messages/messages_vi.properties
books_add=Thêm sách books_details=Chi tiết sách books_edit =Sửa sách
Displaying Badges
For visual clarity, we can also display the number of books and the number of authors directly on the menu by creating a class to add information to the view, for example AdminBookStoreDecorator with content as follows:
@EzySingleton @AllArgsConstructor public class AdminBookStoreViewDecorator implements ViewDecorator { private final AdminBookAuthorService bookAuthorService; private final AdminProductBookService productBookService; @Override public void decorate(HttpServletRequest request, View view) { Reactive.multiple() .register( "book_store.books", productBookService::countAllBooks ) .register( "book_store.authors", bookAuthorService::countAllAuthors ) .blockingConsume(it -> view.putKeyValuesToVariable( VIEW_VARIABLE_MENUITEM_BADGES, it.valueMap() ) ); } }
Then, the corresponding number of books and the number of authors will be displayed on the menu as follows:
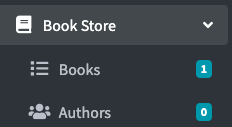
For full example, you can take a look here.