Create Maven Project
Updated at 16856770000001. Introduction
Apache Maven is a software project management and comprehension tool. Based on the concept of a project object model (POM), Maven can manage a project's build, reporting and documentation from a central piece of information. Maven's features include:- Simple project setup that follows best practices.
- Consistent usage across all projects.
- Dependency management including automatic updating.
- A large and growing repository of libraries.
- Extensible, with the ability to easily write plugins in Java or scripting languages.
- Instant access to new features with little or no extra configuration.
- Model-based builds − Maven is able to build any number of projects into predefined output types such as jar, war, metadata.
- Coherent site of project information − Using the same metadata as per the build process, maven is able to generate a website and a PDF including complete documentation.
- Release management and distribution publication − Without additional configuration, maven will integrate with your source control system such as CVS and manages the release of a project.
- Backward Compatibility − You can easily port the multiple modules of a project into Maven 3 from older versions of Maven. It can support the older versions also.
- Automatic parent versioning − No need to specify the parent in the sub module for maintenance.
- Parallel builds − It analyzes the project dependency graph and enables you to build schedule modules in parallel. Using this, you can achieve the performance improvements
of 20-50%.13. Better Error and Integrity Reporting − Maven improved error reporting, and it provides you with a link to the Maven wiki page where you will get full description of the error.
- Execute shell commands from Maven's pom.xml
2.Prerequisite
We will use Java 8, so if you haven’t installed it yet, please download and install it. Download jdk 8 at here:
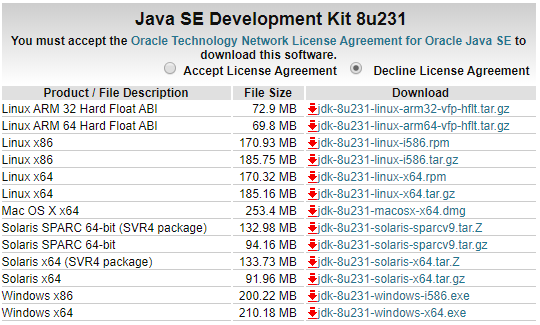
You need select a version for your OS, example me it’s Windows x64. Also, you need setup JAVA_HOME
path variable.
3.Create a maven project on Eclipse
If don’t have Eclipse please download and install Eclipse for JavaEE version
- 1 Open Eclipse and select
File->New->Other
, type text filter tomaven
and select Maven Project.
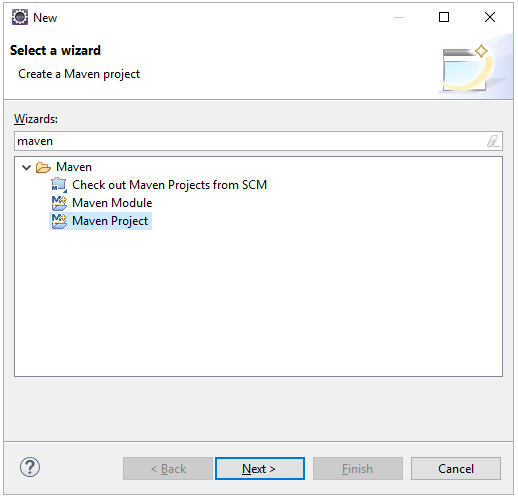
- 2 Click next and we have:
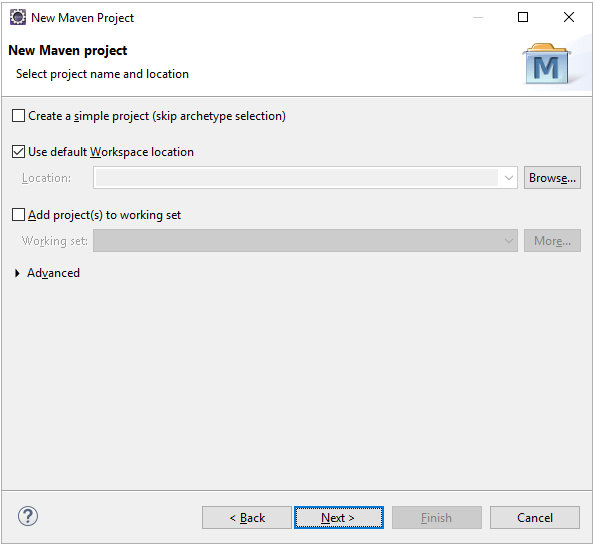
- 3 Click Next and we have:
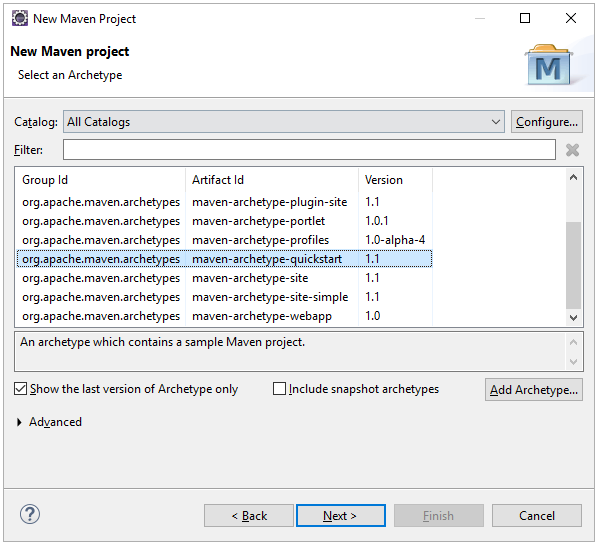
This step allow you select an archetype you want, if you don't want we will use default choice.
- 4 Click next and we have:
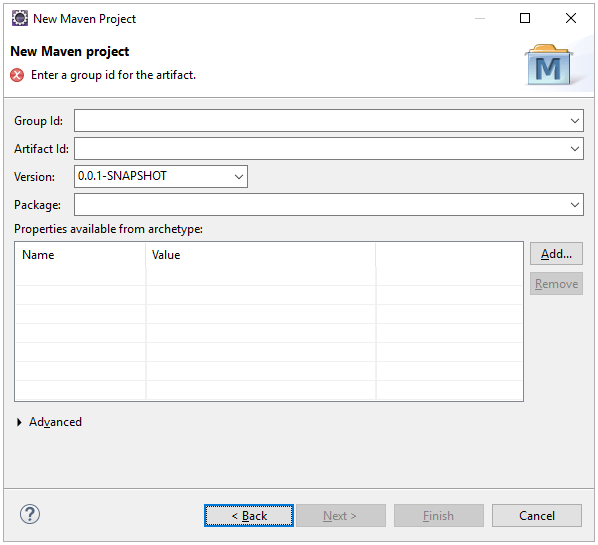
Fill information follow:
Group Id: com.example Artifact Id: hello-world Package: com.example.hello_world
- 5 Click finish and we have a project in eclipse Package Explore:
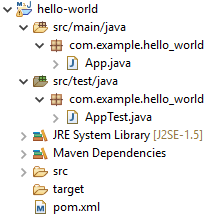
4.Create a maven project on Intellij
If you don’t have Intellij IDE, download here and install it
- 1 Open Intellij IDE and click
New->Project
, we have the dialog:
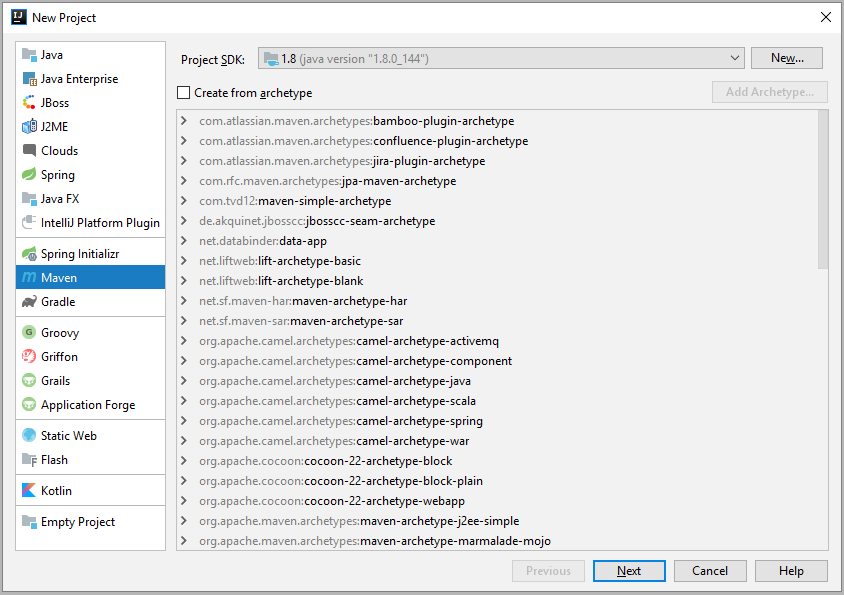
Select Maven on left side, check to Create from archetype
, find and select an archetype that you want, in this case we will select org.apache.maven.archetypes:maven-archetype-quickstart
. Please read this guide to know how to add an archetype.
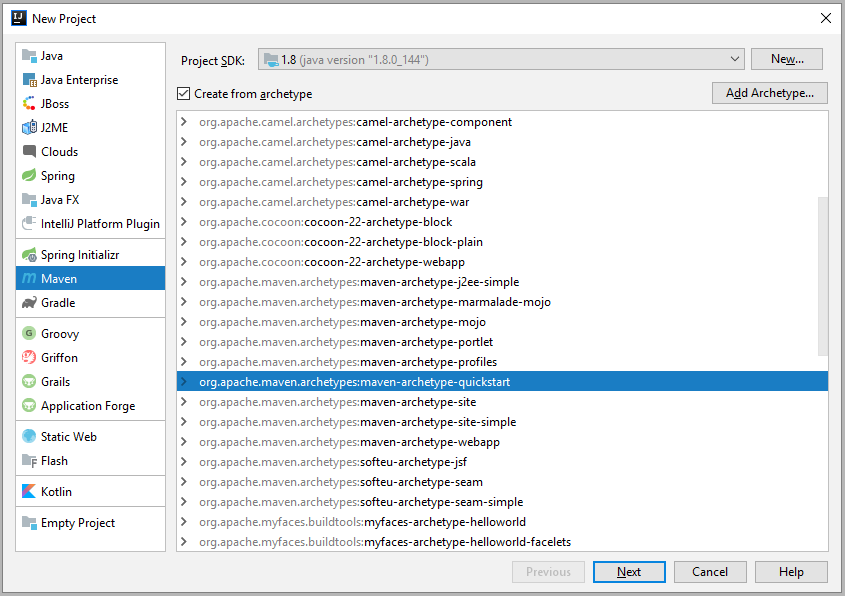
- 2 Click next and we have:
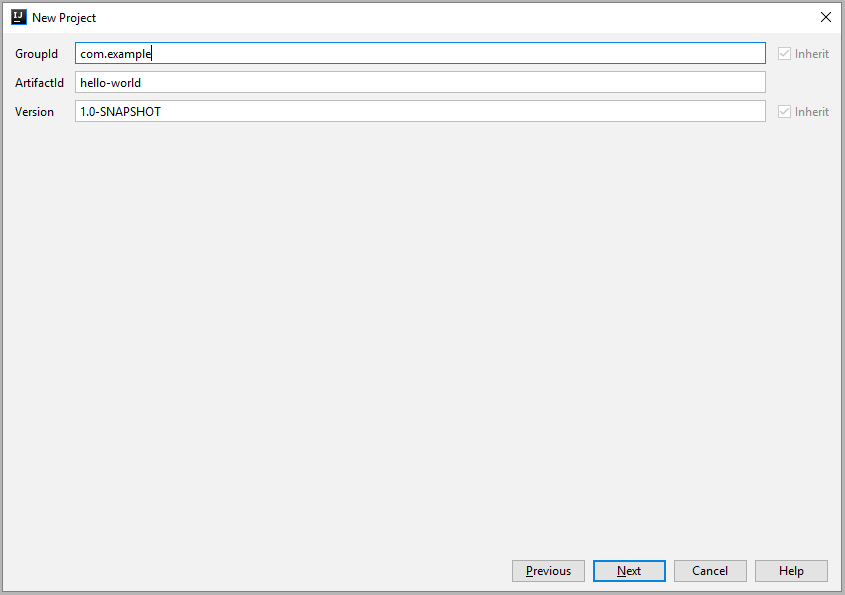
Fill information follow:
com.example ArtifactId: hello-world
- 3 Click Next, Next (double Next) and we have
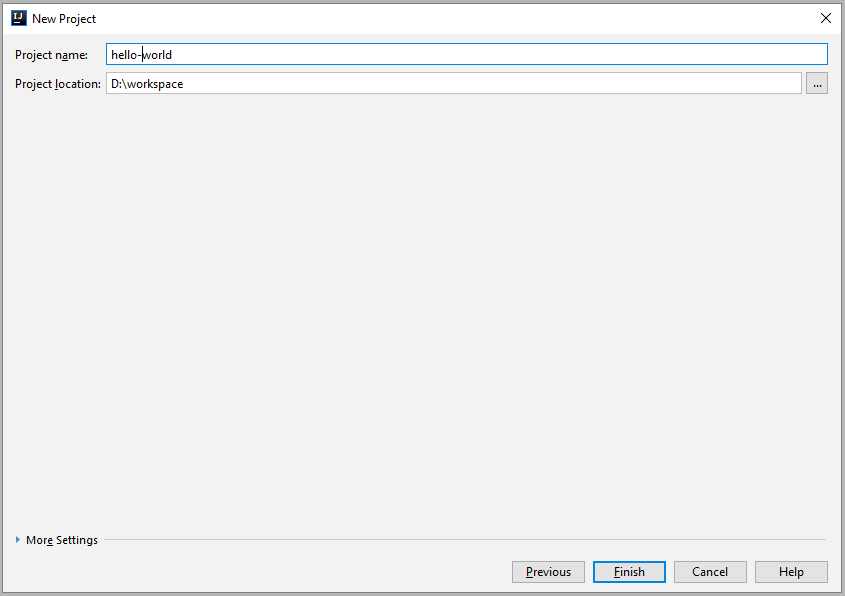
You can change Project name and Project location to fit your purpose
- 4 Click Next (select New Window if the New Project popup open) and we have:
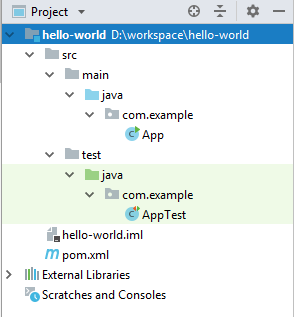
5.Config maven project
5.1 Config pom.xml
Open pom.xml file, we currently have:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>hello-world</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>hello-world</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
By default maven use Java 5, to use Java 8 we need setup for it. In properties tag add 2 properties:
<maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target>
In plugins tag add the plugin:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>${maven.compiler.source}</source> <target>${maven.compiler.target}</target> </configuration> </plugin>
And now we have the pom.xml:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>hello-world</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>hello-world</name> <url>http://maven.apache.org</url> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>${maven.compiler.source}</source> <target>${maven.compiler.target}</target> </configuration> </plugin> </plugins> </build> </project>
On Eclipse, right click to project and select Maven->Update Project
or Alt+F5
, On Intellij right click to project and select Maven->Re-import
and now we have the hello-world
project with Java 8
- 2 Config unitest dependency.
The junit version 3.8.1 is too old, and we want use TestNG and jacoco to check coding coverage, we will replace junit dependency with test-util dependency:
<dependency> <groupId>com.tvd12</groupId> <artifactId>test-util</artifactId> <version>1.1.8</version> </dependency>
Add jacoco dependency:
<plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>0.8.4</version> <executions> <execution> <id>prepare-agent</id> <goals> <goal>prepare-agent</goal> </goals> </execution> <execution> <id>default-report</id> <phase>prepare-package</phase> <goals> <goal>report</goal> </goals> </execution> </executions> </plugin>
And now we have pom.xml
:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>hello-world</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>hello-world</name> <url>http://maven.apache.org</url> <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>com.tvd12</groupId> <artifactId>test-util</artifactId> <version>${testUtitlitiesVersion}</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.1</version> <configuration> <source>${maven.compiler.source}</source> <target>${maven.compiler.target}</target> </configuration> </plugin> <plugin> <groupId>org.jacoco</groupId> <artifactId>jacoco-maven-plugin</artifactId> <version>0.8.4</version> <executions> <execution> <id>prepare-agent</id> <goals> <goal>prepare-agent</goal> </goals> </execution> <execution> <id>default-report</id> <phase>prepare-package</phase> <goals> <goal>report</goal> </goals> </execution> </executions> </plugin> </plugins> <pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.22.2</version> <configuration> <suiteXmlFiles> <suiteXmlFile>src/test/resources/AllTests.tng.xml</suiteXmlFile> </suiteXmlFiles> </configuration> </plugin> </plugins> </pluginManagement> </build> </project>
We get errors at AppTest.java too, don’t worry, change it’s content to:
package com.example.hello_world; import org.testng.annotations.Test; public class AppTest { @Test public void test() { System.out.println("I'm a test"); } }
To build project we need create AllTest.tng.xml in src/test/resources folder with content:
<suite name="AllTests"> <test name="All"> <packages> <package name="com.example.hello_world"></package> </packages> </test> </suite>
Finally, we have hello-world project with structure:
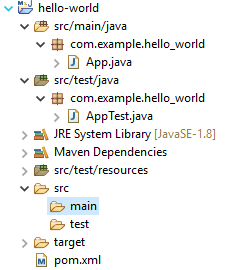
Build project with goals: clean install. Cheer! We done.
6.Conclusion
Use maven to manage project’s build is a good choice, we done need care about library import, and project export, only need add dependencies and build, everything is simple.