Introduce EzyRabbitMQ
Updated at 1689997963000Overview
EzyRabbitMQ (Easy going to RabbitMQ Interaction) is a framework support to interact to RabbitMQ, It supports:- RPC (Remote procedure call)
- Publish and consume message
- Serialize and Deserialize to map message's data in byte array to POJO
- Annotation driven
2. Structure of EzyRabbitMQ
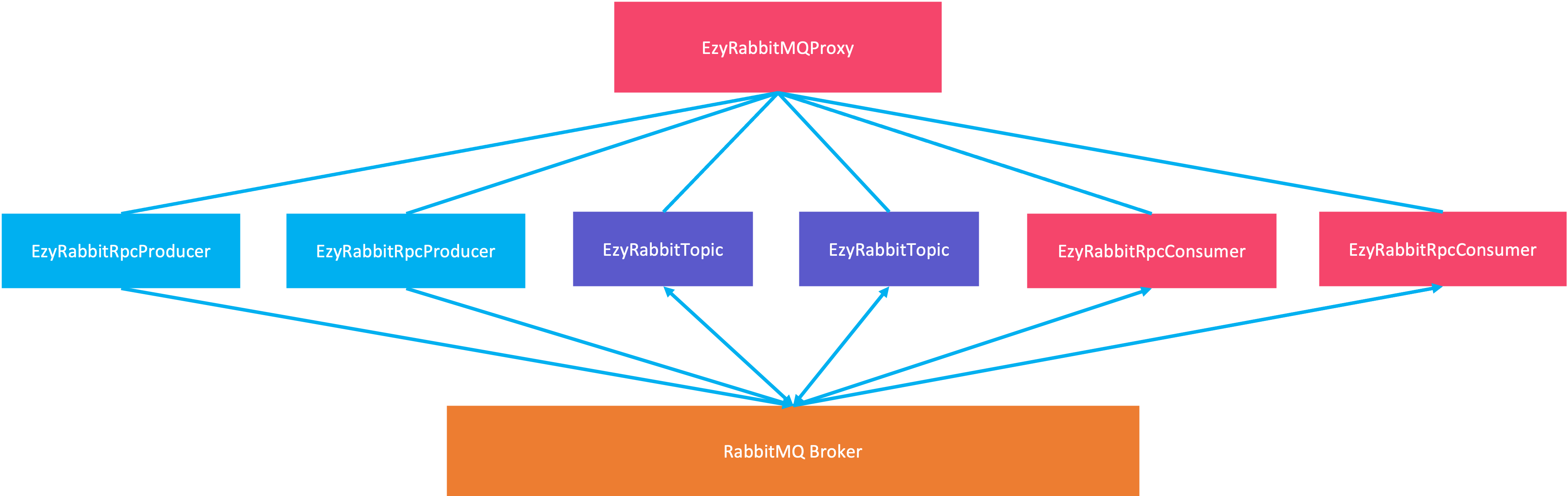
EzyRabbitMQProxy
: Manages producers, consumers, topics and data serializers.EzyRabbiProducer
: Supports to send messages and RPC.EzyRabbitProducer
: Supports to receive, consume the messages and response the RPC resultEzyRabbitTopic
: Supports to send and consume messages via topic
3. Install EzyRabbitMQ
1. To create EzyRabbitMQ we need add dependency
<dependency> <groupId>com.tvd12</groupId> <artifactId>ezymq-rabbitmq</artifactId> <version>1.2.5</version> </dependency>
The latest version can be found in the Maven Central repository. You can configure EzyRabbitMQ like this:
EzyRabbitMQProxy proxy = EzyRabbitMQProxy.builder()
.scan("com.tvd12.ezymq.rabbitmq.test")
.build();
2. You can add to your configuration file like this:
# for application.yaml rabbitmq: producers: test: default consumers: test: default topics: hello: producer: default consumer: default
# for application.properties rabbitmq.producers.test=default rabbitmq.consumers.test=default rabbitmq.topics.hello.producer=default rabbitmq.topics.hello.consumer=default
4. Example
Let’s say we need consume a message to sum 2 integer numbers and get the result, we can do it with EzyRabbitMQ follow by bellow steps.
4.1 Create request/response classes
We need create a request class has 2 fields: a
and b
:
package com.tvd12.ezymq.rabbitmq.test.request; import com.tvd12.ezyfox.message.annotation.EzyMessage; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @Data @EzyMessage @AllArgsConstructor @NoArgsConstructor public class SumRequest { private int a; private int b; }
And a response class has 1 field sum
:
package com.tvd12.ezymq.rabbitmq.test.response; import com.tvd12.ezyfox.message.annotation.EzyMessage; import lombok.AllArgsConstructor; import lombok.Getter; import lombok.ToString; @Getter @EzyMessage @ToString @AllArgsConstructor public class SumResponse { private int sum; }
4.2 Create message handler class
Now we need create a handler class to handle the message:
package com.tvd12.ezymq.rabbitmq.test.handler; import com.tvd12.ezymq.rabbitmq.annotation.EzyActiveHandler; import com.tvd12.ezymq.rabbitmq.handler.EzyActiveRequestHandler; import com.tvd12.ezymq.rabbitmq.test.request.SumRequest; import com.tvd12.ezymq.rabbitmq.test.response.SumResponse; @EzyActiveHandler("sum") public class SumRequestHandler implements EzyActiveRequestHandler { @Override public Object handle(SumRequest request) { return new SumResponse( request.getA() + request.getB() ); } }
4.3 Create a program for rpc client
We need create a class has main
method to start a rpc client:
package com.tvd12.ezymq.rabbitmq.test; import com.tvd12.ezymq.rabbitmq.EzyRabbitMQProxy; import com.tvd12.ezymq.rabbitmq.EzyActiveRpcProducer; import com.tvd12.ezymq.rabbitmq.test.request.SumRequest; import com.tvd12.ezymq.rabbitmq.test.response.SumResponse; public final class SumRpcClientProgram { public static void main(String[] args) { final EzyRabbitMQProxy proxy = EzyRabbitMQProxy .builder() .scan("com.tvd12.ezymq.rabbitmq.test") .build(); final EzyActiveRpcProducer producer = proxy.getRpcProducer("test"); SumResponse sumResponse = producer.call( "sum", new SumRequest(1, 2), SumResponse.class ); System.out.println("sum result: " + sumResponse); } }
With configration like this:
# for application.yaml rabbitmq: producers: test: default
4.4 Create a program for rpc server
We need create a class has main
method to start consumers:
package com.tvd12.ezymq.rabbitmq.test; import com.tvd12.ezymq.rabbitmq.EzyRabbitMQProxy; public final class SumRpcServerProgram { public static void main(String[] args) { EzyRabbitMQProxy .builder() .scan("com.tvd12.ezymq.rabbitmq.test") .build(); } }
With configration like this:
# for application.yaml rabbitmq: consumers: test: default
4.5 Run the program
Please make sure you have downloaded, installed, and started the RabbitMQ broker.
Now run the both SumRpcServerProgram
and SumRpcClientProgram
programs separately, we will see the log in the SumRpcClientProgram
:
sum result: SumResponse(sum=3)
Next step
You can see how to configure.