EzyHTTP Upload file
Updated at 1685686579000Same as every HTTP server framewords, EzyHttp allows you upload file, too. And we recommend you let's use asynchronous API, to know why, please take a look the explanation here.
1. FileUploader
EzyHTTP provides to you an convenient FileUploader
class. It wraps ResourceUploadManager
, an event loop like below:
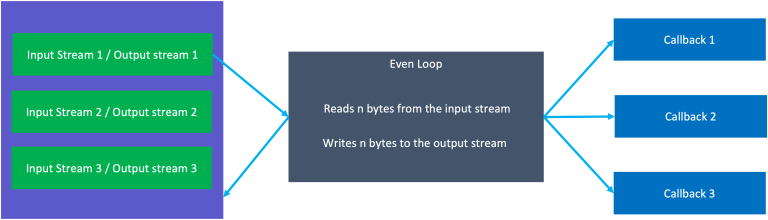
And the event loop will do like this:
loop: take a inputstream/outputstream pair from the queue: take 1024 bytes from the inputstream of the requested file and push to client's outputstream if there is no remain data in the inputstream then response to the client: call to the callback and done else push the the inputstream/outputstream pair back to the queue
2. Enable file upload configuration
To enable file upload, you can add the configuration to your application.properties file like this:
resources.upload.enable=true
If you want to set queue capacity and thread pool size, buffer size, you can setup:
resources.upload.capacity=[an integer value] resources.upload.thread_pool_size=[an integer value] resources.upload.buffer_size=[an integer value]
3. Upload file asynchronously
To upload a file asynchronously with FilerUploader, you can do like this:
@Controller("/api/v1") @AllArgsConstructor public class FileController { private final FileUploadService fileUploadService; @Async @DoPost("/files/upload") public void uploadPost( RequestArguments requestArguments ) throws Exception { fileUploadService.accept(requestArguments.getRequest()); } } @EzySingleton @AllArgsConstructor public class FileUploadService { private final FileUploader fileUploader; public void accept(HttpServletRequest request) throws Exception { accept( request, request.getPart("file"), () -> System.out.println("Upload finished") ); } public void accept( HttpServletRequest request, Part part, EzyExceptionVoid callback ) { String fileName = part.getSubmittedFileName(); File file = new File("files/" + fileName); AsyncContext asyncContext = request.getAsyncContext(); fileUploader.accept(asyncContext, part, file, callback); } }
4. Upload file Synchronously
To upload a file synchronously, you can do like this:
@Controller("/api/v1") public class FileController { @DoPost("/files/upload-sync") public ResponseEntity uploadSyncPost( HttpServletRequest request ) throws Exception { Part part = request.getPart("file"); String fileName = part.getSubmittedFileName(); File file = new File("files/" + fileName); InputStream inputStream = part.getInputStream(); byte[] buffer = new byte[1024]; try (OutputStream outputStream = new FileOutputStream(file)) { while (true) { int read = inputStream.read(buffer); if (read > 0) { outputStream.write(buffer, 0, read); } else { break; } } } return ResponseEntity.noContent(); } }
Next step
You can see How to download a file.