Introduce EzyFox Binding
Updated at 16858085470001. Structure of EzyFox Binding
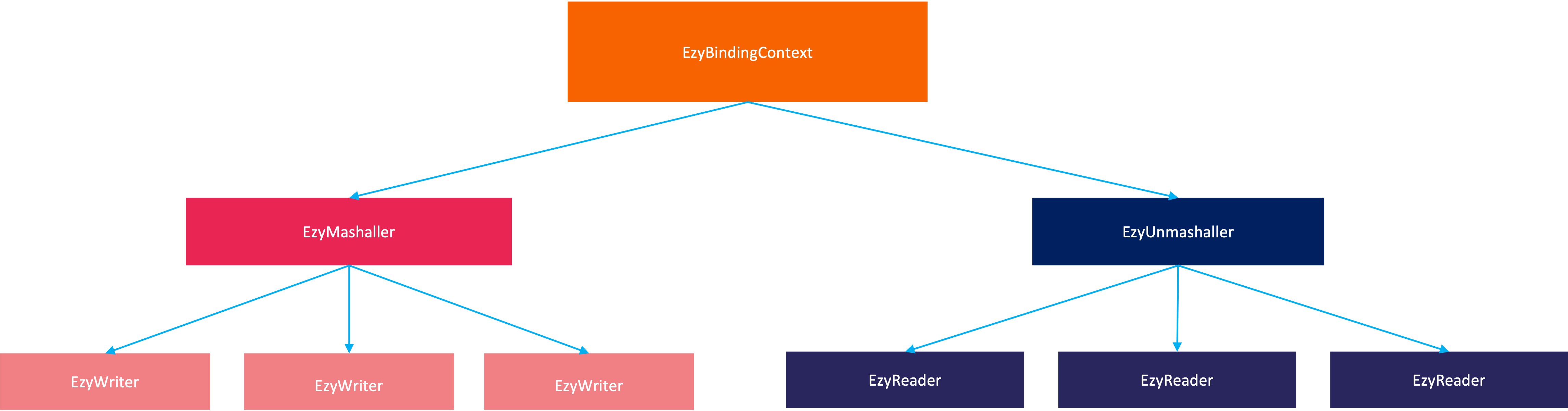
- EzyBindingContext: a composite object, it creates EzyMashaller and EzyUnmashaller object
- EzyMashaller: a composite object, it manages EzyWriter objects
- EzyUnmashaller: a composite object, it manages EzyReader objects
- EzyWriter: convert a POJO to EzyArray or EzyObject
- EzyReader: convert a EzyArray or EzyObject to POJO
EzyFox Binding also has many annotations and you can find out theme here.
2. Install EzyFox Binding
You can add to your dependency like this:
<dependency> <groupId>com.tvd12</groupId> <artifactId>ezyfox-binding</artifactId> <version>1.2.9</version> </dependency>
The latest version can be found in the Maven Central repository.
3. Create a EzyBindingContext
To create a EzyBindingContext you just need provide packages and EzyFox Binding will scan and create EzyReader, EzyWriter for which classes that's annotated by @EzyArrayBinding
or @EzyObjectBinding
annotations, example:
final EzyBindingContext bindingContext = EzyBindingContext.builder() .scan("com.tvd12.ezyfox.example.binding") .build();
4. Marshal an POJO
To marshal a POJO you need create a EzyMarshaller instance first, please remember you just need create only one EzyMarshaller instance. And then use marshal
function to do. For which class's annotated with @EzyArrayBinding
, it will be marshalled to EzyArray. For which class's annotated with @EzyObjectBinding
, it will be marshalled to EzyObject, example:
package com.tvd12.ezyfox.example.binding.data; import com.tvd12.ezyfox.binding.annotation.EzyArrayBinding; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @Data @EzyArrayBinding @AllArgsConstructor @NoArgsConstructor public class Author { private long id; private String name; }
package com.tvd12.ezyfox.example.binding.data; import com.tvd12.ezyfox.binding.annotation.EzyObjectBinding; import lombok.AllArgsConstructor; import lombok.Data; import lombok.NoArgsConstructor; @Data @EzyObjectBinding @AllArgsConstructor @NoArgsConstructor public class Book { private long id; private String name; private long authorId; }
final EzyMarshaller marshaller = bindingContext.newMarshaller(); final EzyObject bookMap = marshaller .marshal(new Book(1, "EzyFox in Action", 1)); System.out.println("bookMap: " + bookMap); final EzyArray authorArray = marshaller .marshal(new Author(1, "Young Monkeys")); System.out.println("authorArray: " + authorArray);
5. Unmarshal a value
To unmarshal a value to POJO you need create a EzyUnmarshaller instance first, please remember you just need create only one EzyUnmarshaller instance. And then use unmarshal
function to do. For which class's annotated with @EzyArrayBinding
, it will be unmarshalled form a EzyArray. For which class's annotated with @EzyObjectBinding
, it will be unmarshalled form a EzyObject, example:
final EzyUnmarshaller unmarshaller = bindingContext.newUnmarshaller(); final Book book = unmarshaller.unmarshal(bookMap, Book.class); System.out.println("book: " + book); final Author author = unmarshaller.unmarshal(authorArray, Author.class); System.out.println("author: " + author);