Introduce EzyFox
Updated at 1699803592000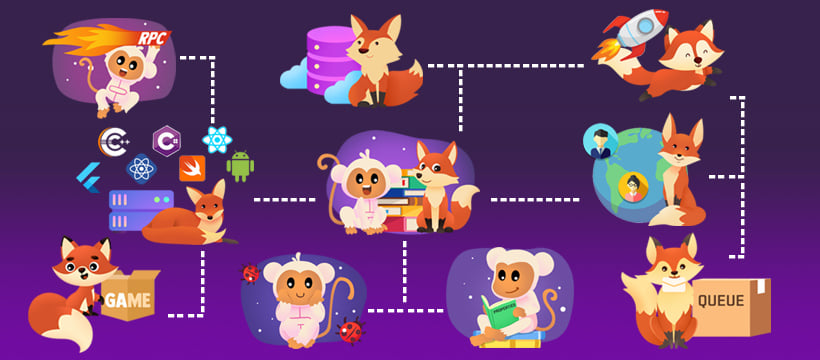
Generics with EzyFox
Java provides collections framework to support Generics to specify the type of objects stored in a collection instance. EzyFox provides some ways to work with Java Collections to create an ArrayList.
List stringList = Lists.newArrayList("Hello", "World");
Create a MultiMap:
EzyMapSet multimap = new EzyHashMapSet<>(); multimap.addItems("Hello", 1, 2, 3); multimap.addItems("World", 4, 5, 6);
Reflection with EzyFox
Reflection) allows programmer interact to package, class, method and field via name and you done need care about type. Reflection is usually used to boot up an Application. Because it's too slow so it usually combines with JIT to make powerfulCreate an Instance
Object string = EzyClasses.newInstance("java.lang.String");
Get methods of a class
EzyClass stringClass = new EzyClass(String.class);
List stringMethods = stringClass.getDeclaredMethods();
Get Generics argument of return type
Method getStringsMethod = EzyMethods.getMethod(EzyReflectionExample.class, "getStrings"); Type genericReturnType = getStringsMethod.getGenericReturnType(); Class genericClass = EzyGenerics.getOneGenericClassArgument(genericReturnType); System.out.println("genericClass: " + genericClass);
Concurrency with EzyFox
Concurrency allows multi tasks run on multi threads and from version 1.6 Java's provided the java.util.concurrent package to support Concurrency Programming in many ways and EzyFox's provided to create a Thread Pool.
ExecutorService threadPool = EzyExecutors.newFixedThreadPool(3, "test-thread-name");
Work with Future
EzyFuture future = new EzyFutureTask(); Thread newThread = new Thread(() -> { future.setResult("Hello World"); }); newThread.start(); String result = future.get();
Work with Lock
EzyMapLockProvider lockProvider = new EzyHashMapLockProxyProvider(); Lock lock = lockProvider.provideLock("test"); lock.lock(); try { // do something } finally { lock.unlock(); lockProvider.removeLock("test"); }