EzyFox CSharp Client SDK
Updated at 1691242894000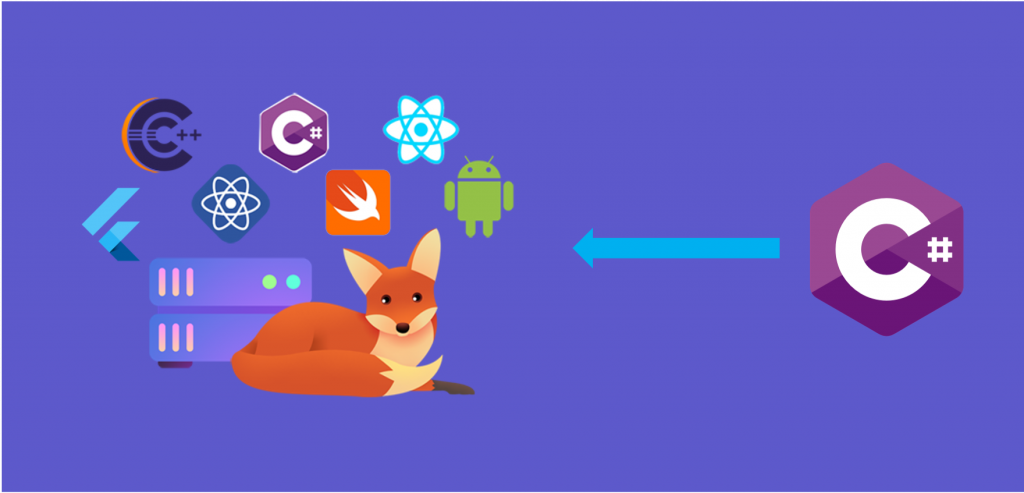
1. Introduce
EzyFox CSharp SDK is a csharp client library of EzyFox Server. It supports tcp protocol and use MessagePack for data transportation. You can use this SDK for every project written in CSharp. It's free available and open source on Github.2. Installation
Clone ezyfox-server-android-client to your project:
git clone https://github.com/youngmonkeys/ezyfox-server-csharp-client.git
With Unity you can clone to Assets folder.
3. Coding
Completed source code available on space shooter example.
3.1 Create a configuration
Please take a look EzyClientConfig file if you want to see more configurable fields:
var config = EzyClientConfig.builder() .clientName(ZONE_NAME) .build(); }
If you want to enable data encryption, you can add to the config .enableSSL()
:
var config = EzyClientConfig.builder() .clientName(ZONE_NAME) .enableSSL() .build(); }
3.2 Create a Client
After create the configuration, you can create a client like this. Take a look EzyClient and EzyClients to get more information:
EzyClient client = new EzyTcpClient(clientConfig);
clients.addClient(client);
And if you want to create a client for both TCP and UDP, you can use
socketClient = new EzyUTClient(config);
EzyClients.getInstance().addClient(socketClient);
3.3 Setup the Client
After create the Client, let's setup it, 'setup' mean add event handlers and data handlers. List of event handlers include:
- CONNECTION_SUCCESS: Fire when the client connected to server
- CONNECTION_FAILURE: Fire when the client connect to server failed
- DISCONNECTION: Fire when the client was disconnected from server
- LOST_PING: Fire when client send ping but didn't received pong command from server
- TRY_CONNECT: Fire when the client connect to server failed and retry to connect again
Take a look EzyEventType to get more information.
List of data commands include:
- ERROR: Fire when the client received and error from server
- HANDSHAKE: Client send and receive handshake command
- PING: Client send ping command to keep connection
- PONG: Fire when client received pong response from server
- LOGIN: Client send and receive login command if login successfully
- LOGIN_ERROR: Fire when received login error response from server
- APP_ACCESS: Client send this command to join to an application and receive when join successfully
- APP_REQUEST: Client send data to an application on server and receive if there is any response
- APP_EXIT: Client send to server to exit from an application
- APP_ACCESS_ERROR: Fire when received join an application failed from server,
- APP_REQUEST_ERROR: Fire when received an error response from application on server,
- PLUGIN_INFO: Client send to get information of a plugin and receive response from server,
- PLUGIN_REQUEST: lient send data to an plugin on server and receive if there is any response
Take a look EzyCommand to get more information.
You can setup the client like this:
var setup = socketClient.setup(); setup.addEventHandler(EzyEventType.CONNECTION_SUCCESS, new EzyConnectionSuccessHandler()); setup.addEventHandler(EzyEventType.CONNECTION_FAILURE, new EzyConnectionFailureHandler()); setup.addEventHandler(EzyEventType.DISCONNECTION, new DisconnectionHandler()); setup.addDataHandler(EzyCommand.HANDSHAKE, new HandshakeHandler()); setup.addDataHandler(EzyCommand.LOGIN, new LoginSuccessHandler()); setup.addDataHandler(EzyCommand.LOGIN_ERROR, new EzyLoginErrorHandler()); setup.addDataHandler(EzyCommand.APP_ACCESS, new AppAccessHandler()); setup.addDataHandler(EzyCommand.UDP_HANDSHAKE, new UdpHandshakeHandler());
Follow by the authentication flow:
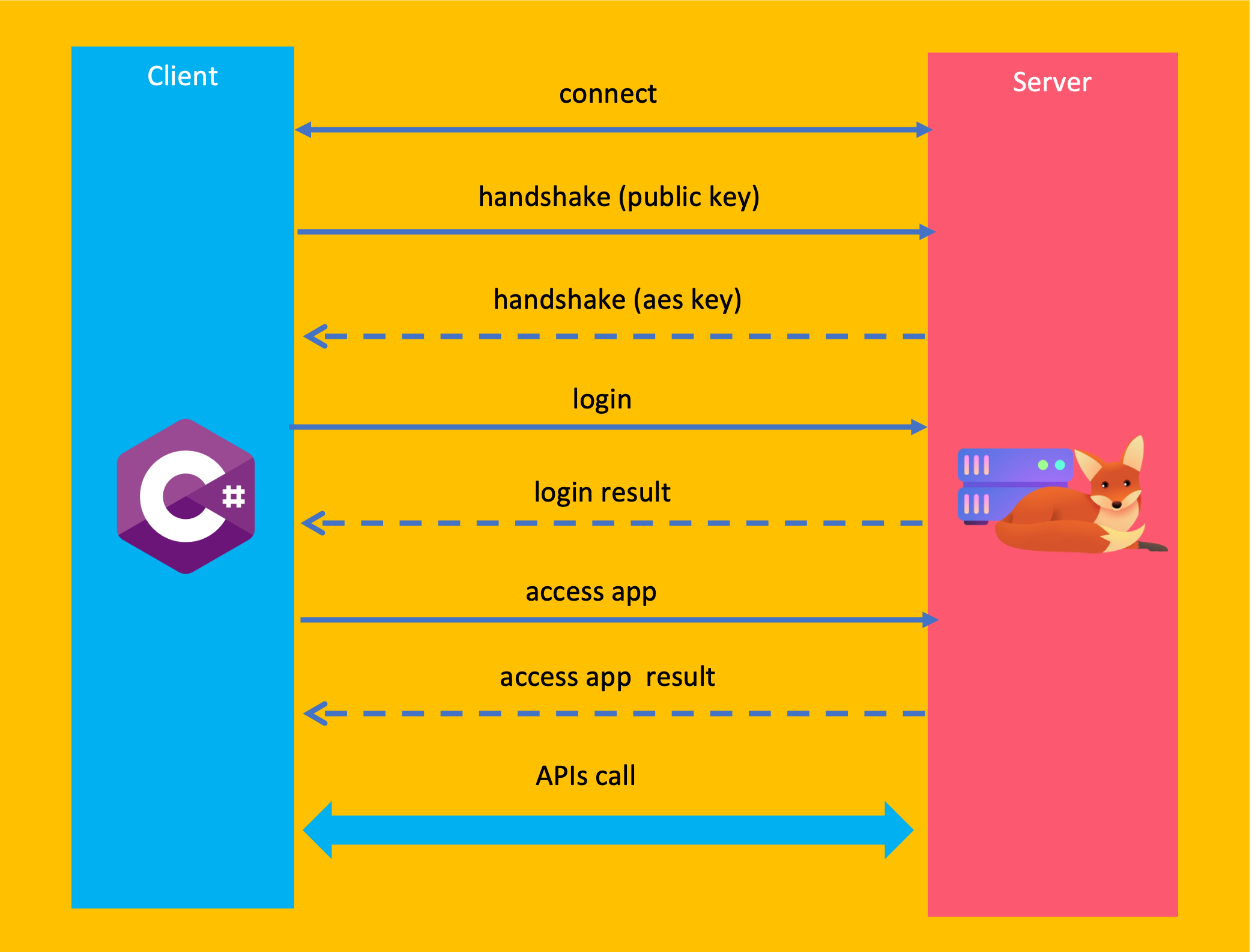
3.4 Customer an event handler
You can customer to do everything you want, please take a look handler package to see default event handlers and take a look to this file for full example.
class DisconnectionHandler : EzyDisconnectionHandler { protected override void postHandle(EzyDisconnectionEvent evt) { SocketClientProxy.getInstance().emitDisconnected(); } }
3.5 Custom a data handler
You must custom to handle list of commands:
- HANDSHAKE: to send LOGIN command
- LOGIN: to send APP_ACCESS or PLUGIN_INFO command
You should custom to handle list of commands:
- LOGIN_ERROR: Maybe to show a notification to user
- APP_ACCESS: Maybe to trigger something and allow user interact your application
Please take a look handler package to get default data handlers and this file for full example
class DisconnectionHandler : EzyDisconnectionHandler { protected override void postHandle(EzyDisconnectionEvent evt) { SocketClientProxy.getInstance().emitDisconnected(); } }
3.6 Setup an App
To listen and process response data of an application received from server you need setup to register handler mapped to command like this:
var appSetup = setup.setupApp(APP_NAME); appSetup.addDataHandler("reconnect", new ReconnectResponseHandler()); appSetup.addDataHandler("getGameId", new GetGameIdResponseHandler()); appSetup.addDataHandler("startGame", new StartGameResponseHandler());
3.7 Connect to server
After setup the client and an app, it's time to connect to server
client.connect(host, 3005)
3.8 Send a request to server
After connect to server and access an app successfully (you need ensure by handle APP_ACCESS response), you can allow interact and send a request to server
var request = EzyEntityFactory.newObject(); request.put("gameName", GAME_NAME); request.put("gameId", gameId); socketClient.getApp().send("startGame", request);
And if you have accessed many application in the client, you must get the app you want to send a request
var zone = socketClient.getZone(); if(zone != null) { var app = zone.getAppManager().getAppByName("appName"); if(app != null) { var request = EzyEntityFactory.newObject(); request.put("gameName", GAME_NAME); request.put("gameId", gameId); app.send("startGame", request); } }
Please take a look EzyApp to see how an app send a request to server, look EzySimpleAppManager to see how to get an app. For full example please take a look this file
4.With Unity
4.1 Config logger
With Unity, we need a logger class to view Ezyfox Unity client SDK's log like this:
using System; using UnityEngine; using com.tvd12.ezyfoxserver.client.logger; public class UnityLogger : EzyLogger { private readonly object type; public UnityLogger(object type) { this.type = type; } public void debug(string format, params object[] args) { Debug.Log(type + " - " + format); } // other methods }
For full source code, please refer UnityLogger class We need set this logger class to EzyLoggerFactory in entry point class like Done\_GameController like this
EzyLoggerFactory.setLoggerSupply(type => new UnityLogger(type));
4.2 Loop to process socket events
To process events from socket, you need create a empty object and add a script contain MonoBehaviour like this
using UnityEngine; public class GameLoop : MonoBehaviour { private void Update() { SocketClientProxy.getInstance().processEvents(); } }
For processEvents
your can refer SocketClientProxy class