EzyFox Flutter Client SDK
Updated at 1699804322000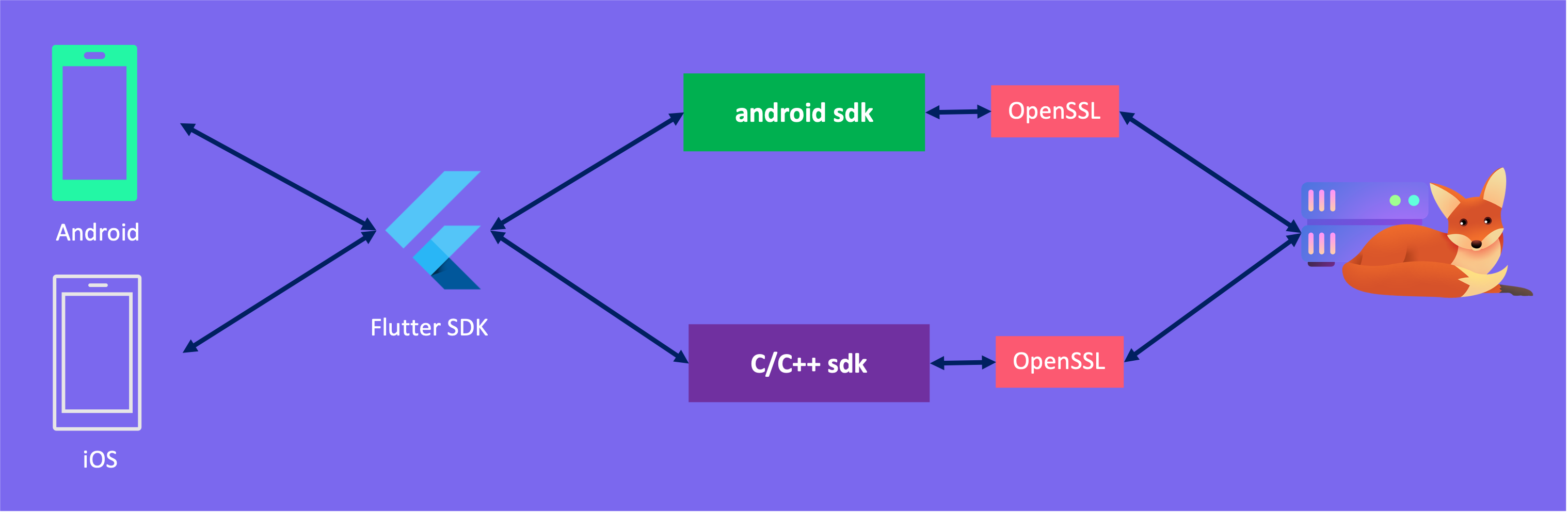
1. Introduce
EzyFox Flutter SDK is a Swift client library of EzyFox Server. It supports TCP protocol and use MessagePack for data transportation. You can use this SDK for every projects use Swift (i.e iOS, apple desktop apps). It's free available and open source on Github2. Installation
Clone ezyfox-server-flutter-client
to your project
git clone --recurse-submodules https://github.com/youngmonkeys/ezyfox-server-flutter-client.git ezyfox_server_flutter_client
Update pubspec.yaml
Open pubspec.yaml
file and update:
dependencies: flutter: sdk: flutter ezyfox_server_flutter_client: path: ./ezyfox_server_flutter_client
Setup Android
Open settings.gradle
file and add (you can refer here for full example):
include ':socket' project(':socket').projectDir = new File('../ezyfox_server_flutter_client/android')
Setup iOS
Drag and drop folder ezyfox_server_flutter_client/ios/EzyClient
to your XCode and we have:
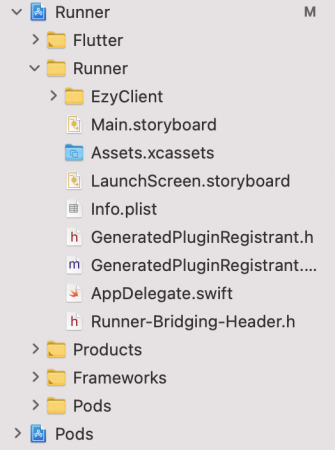
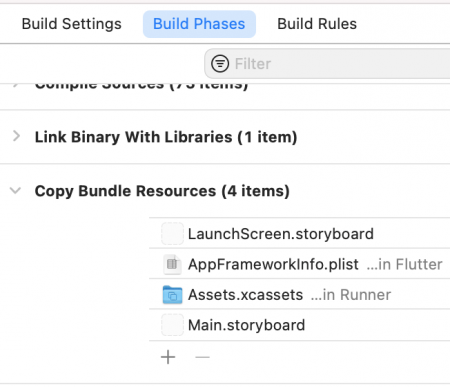
Open AppDelegate.swift file and add to application
method:
let controller : FlutterViewController = window?.rootViewController as! FlutterViewController EzyClientProxy.getInstance().registration(controller.binaryMessenger)
Open Runner-Bridging-Header.h file and add:
#import "EzyClientProxy.h" #import "EzyEncryptionProxy.h"
3. Coding
Completed source code avalable on Flutter Example, class SocketProxy
3.1 Import
import 'package:ezyfox_server_flutter_client/ezy_client.dart'; import 'package:ezyfox_server_flutter_client/ezy_clients.dart'; import 'package:ezyfox_server_flutter_client/ezy_config.dart'; import 'package:ezyfox_server_flutter_client/ezy_constants.dart'; import 'package:ezyfox_server_flutter_client/ezy_entities.dart'; import 'package:ezyfox_server_flutter_client/ezy_handlers.dart';
3.2 Create a configuration
Please take a look EzyConfig class if you want to see more configurable fields
EzyConfig config = EzyConfig(); config.clientName = ZONE_NAME;
3.2 Create a Client
After create the configuration, you can create a client like this. Take a look EzyClient and EzyClients to get more information
EzyClients clients = EzyClients.getInstance(); _client = clients.newDefaultClient(config);
3.3 Setup the Client
After create the client, let's setup it, 'setup' mean add event handlers and data handlers to the client. List of event handlers include:
- CONNECTION_SUCCESS: Fire when the client connected to server
- CONNECTION_FAILURE: Fire when the client connect to server failed
- DISCONNECTION: Fire when the client was disconnected from server
- LOST_PING: Fire when client send ping but didn't received pong command from server
- TRY_CONNECT: Fire when the client connect to server failed and retry to connect again
Take a look EzyConstants class to get more information. List of data commands include:
- ERROR: Fire when the client received and error from server
- HANDSHAKE: Client send and receive handshake command
- PING: Client send ping command to keep connection
- PONG: Fire when client received pong response from server
- LOGIN: Client send and receive login command if login successfully
- LOGIN_ERROR: Fire when received login error response from server
- APP_ACCESS: Client send this command to join to an application and receive when join successfully
- APP_REQUEST: Client send data to an application on server and receive if there is any response
- APP_EXIT: Client send to server to exit from an application
- APP_ACCESS_ERROR: Fire when received join an application failed from server,
- APP_REQUEST_ERROR: Fire when received an error response from application on server,
- PLUGIN_INFO: Client send to get information of a plugin and receive response from server,
Take a look ezy\_constants.dart file and ezy\_handlers.dart file to get more information and you can setup the client like this:
_client.setup.addEventHandler(EzyEventType.DISCONNECTION, _DisconnectionHandler(_disconnectedCallback!)); _client.setup.addEventHandler(EzyEventType.CONNECTION_FAILURE, _ConnectionFailureHandler(_disconnectedCallback!)); _client.setup.addDataHandler(EzyCommand.HANDSHAKE, _HandshakeHandler()); _client.setup.addDataHandler(EzyCommand.LOGIN, _LoginSuccessHandler()); _client.setup.addDataHandler(EzyCommand.APP_ACCESS, _AppAccessHandler());
For TCP we need follow by the authenticaiton flow
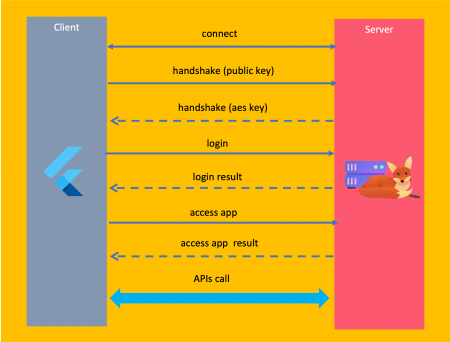
3.4 Customer an event handler
You can custom to do everything you want, please take a look ezy\_handlers.dart file to see default event handlers and take a look to this file for full example.
class _DisconnectionHandler extends EzyDisconnectionHandler { late Function _callback; _DisconnectionHandler(Function callback) { _callback = callback; } @override void postHandle(Map event) { _callback(); } }
3.5 Custom a data handler
You must custom to handle list of commands:
- HANDSHAKE: to send Login command
- LOGIN: to send AppAccess or PluginInfo command
You should custom to handle list of commands:
- LOGIN_ERROR: Maybe to show a notification to user
- APP_ACCESS: Maybe to trigger something and allow user interact your application
Please take a look ezy\_handlers.dart file to get default data handlers and this file for full example
class _HandshakeHandler extends EzyHandshakeHandler { @override List getLoginRequest() { var request = []; request.add(ZONE_NAME); request.add(SocketProxy.getInstance().username); request.add(SocketProxy.getInstance().password); request.add([]); return request; } } class _LoginSuccessHandler extends EzyLoginSuccessHandler { @override void handleLoginSuccess(responseData) { client.send(EzyCommand.APP_ACCESS, [APP_NAME]); } }
3.6 Setup an App
To listen and process response data of an application received from server you need setup to register handler mapped to command like this:
var appSetup = _client.setup.setupApp(APP_NAME); appSetup.addDataHandler("greet", _GreetResponseHandler((message) { _greetCallback!(message); })); appSetup.addDataHandler("secureChat", _SecureChatResponseHandler((message) { _secureChatCallback!(message); }));
3.7 Connect to server
After setup the client and an app, it's time to connect to server
this._client.connect("localhost", 3005);
3.8 Send a request to server
After connect to server and access an app successfully (you need ensure by handle APP_ACCESS response), you can allow interact and send a request to server.
var data = Map(); data["who"] = "Flutter's developer"; app.send("command", data);
And if you have accessed many application in the client, you must get the app you want to send a request
var app = EzyClients.getInstance() .getDefaultClient() .zone! .appManager .getAppByName("appName"); app.send("command", data);
Please take a look EzyApp class to see how an app send a request to server, look EzyAppManager to see how to get an app. For full example please take a look this file