EzyFox Server React Native client SDK
Updated at 1699804355000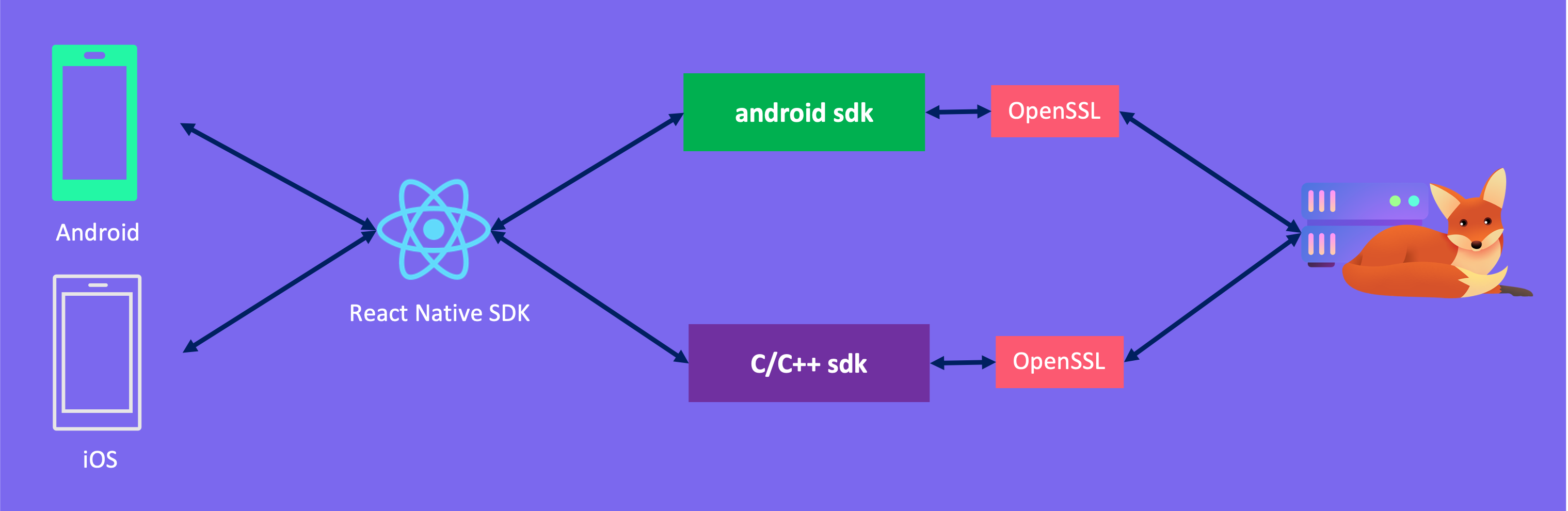
1. Introduce
EzyFox React Native SDK is a javascript client library of EzyFox Server. It supports websocket protocol and use json for data transportation. You can use this SDK for every projects use React Native. It's free available on npm and open source on Github2. Installation
npm i ezyfox-server-react-native-client
3. Coding
Completed source code available on Hello React Native example
3.1 Import
import Ezy from 'ezyfox-server-react-native-client';
3.2 Create a configuration
Please take a look ezy-configs.js file if you want to see more configurable fields
let config = new Ezy.ClientConfig; config.clientName = "clientName"
3.2 Create a Client
After create the configuration, you can create a client like this. Take a look ezy-client.js and ezy-clients.js to get more information
let clients = Ezy.Clients.getInstance(); let client = clients.newClient(config);
3.3 Setup the Client
After create the Client, let's setup it, 'setup' mean add event handlers and data handlers. List of event handlers include:
- CONNECTION_SUCCESS: Fire when the client connected to server
- CONNECTION_FAILURE: Fire when the client connect to server failed
- DISCONNECTION: Fire when the client was disconnected from server
- LOST_PING: Fire when client send ping but didn't received pong command from server
- TRY_CONNECT: Fire when the client connect to server failed and retry to connect again
List of data commands include:
- ERROR: Fire when the client received and error from server
- HANDSHAKE: Client send and receive handshake command
- PING: Client send ping command to keep connection
- PONG: Fire when client received pong response from server
- LOGIN: Client send and receive login command if login successfully
- LOGIN_ERROR: Fire when received login error response from server
- APP_ACCESS: Client send this command to join to an application and receive when join successfully
- APP_REQUEST: Client send data to an application on server and receive if there is any response
- APP_EXIT: Client send to server to exit from an application
- APP_ACCESS_ERROR: Fire when received join an application failed from server,
- APP_REQUEST_ERROR: Fire when received an error response from application on server,
- PLUGIN_INFO: Client send to get information of a plugin and receive response from server,
- PLUGIN_REQUEST: lient send data to an plugin on server and receive if there is any response
Take a look ezy-constants.js to get more information.
You can setup the client like this:
let setup = client.setup;
setup.addEventHandler(Ezy.EventType.DISCONNECTION, disconnectionHandler);
setup.addDataHandler(Ezy.Command.HANDSHAKE, handshakeHandler);
setup.addDataHandler(Ezy.Command.LOGIN, loginSuccessHandler);
setup.addDataHandler(Ezy.Command.LOGIN_ERROR, loginErrorHandler);
setup.addDataHandler(Ezy.Command.APP_ACCESS, accessAppHandler);"
Follow by authentication flow
3.4 Customer an event handler
You can custom to do everything you want, please take a look ezy-event-handlers.js to see default event handlers and take a look to this file for full example.
let disconnectionHandler = new Ezy.DisconnectionHandler(); disconnectionHandler.preHandle = function(event) { // process disconnection event };
3.5 Custom a data handler
You must custom to handle list of commands:
- HANDSHAKE: to send LOGIN command
- LOGIN: to send APP_ACCESS or PLUGIN_INFO command
You should custom to handle list of commands:
- LOGIN_ERROR: Maybe to show a notification to user
- APP_ACCESS: Maybe to trigger something and allow user interact your application
Please take a look ezy-data-handlers.js to get default data handlers and this file for full example
let handshakeHandler.getLoginRequest = function() { let connection = models.connection; let username = connection.username; let password = connection.password; return ["zoneName", username, password, []]; }; let loginSuccessHandler = new Ezy.LoginSuccessHandler(); loginSuccessHandler.handleLoginSuccess = function() { let accessAppRequest = ["appName", []]; this.client.sendRequest(Ezy.Command.APP_ACCESS, accessAppRequest); };
3.6 Setup an App
To listen and process response data of an application received from server you need setup to register handler mapped to command like this:
let setupApp = setup.setupApp("appName"); setupApp.addDataHandler("command", function(app, data) { // handle response app data });
3.7 Connect to server
After setup the client and an app, it's time to connect to server
let url = "ws://localhost:2208/ws"; // replace by your server url
let client = this.getClient();
client.connect(url);
3.8 Send a request to server
After connect to server and access an app successfully (you need ensure by handle APP_ACCESS response), you can allow interact and send a request to server
let zone = this.getClient().zone;
let appManager = zone.appManager;
let app = appManager.getApp();
app.sendRequest("requestCommand", requestData);
And if you have accessed many application in the client, you must get the app you want to send a request
let zone = this.getClient().zone; let appManager = zone.appManager; let app = appManager.getAppByName("appName"); app.sendRequest("requestCommand", requestData);
Please take a look ezy-entities.js to see how an app send a request to server, look ezy-managers.js to see how to get an app. For full example please take a look this file